06. DIV
November 11, 2023About 4 min
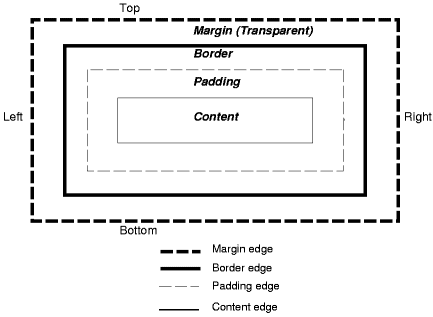
div {
width: 800px;
min-width: 800px;
max-width: 1000px;
height: 800px;
min-height: 800px;
max-height: 1000px;
}
Margin 塌陷问题
.outer {
overflow: hidden; /* scroll / visible */
padding: 1px;
border: 1px;
}
Box
Border
<!doctype html>
<html>
<head>
<style>
/*
width/height stands for the attributes of content
Box style:
border-width
default:3 pixels
Four values:T R B L
Three values:T LR B
Two values:TB LR
border-xxx-width: Set the width of border for different direction. xxx can be set as top/bottom/left/right
border-xxx-color: The same usage with width for specifing color
border-xxx-style: The same usage with width for specifing border style
solid: solid line
dotted: dotted line
dashed: dashed line
double: double solid line
Abbreviation for attribution of border, no order required.
border: 10px solid red;
border-top: 10px solid red;
*/
.box1 {
width: 200px;
height: 200px;
background-color: #bfa;
border: 10px solid red;
border-right: none;
}
</style>
</head>
<body>
<div class="box1"></div>
</body>
</html>
Padding
<!doctype html>
<html>
<head>
<style>
/*
(padding)
padding-top
padding-right
padding-bottom
padding-left
-- background color will extend to padding area. The size of box is defined by content, padding, and border.
The abbreviation of padding keep the same with border.
*/
.box1 {
width: 200px;
height: 200px;
background-color: #bfa;
border: 10px solid red;
border-right: none;
padding: 20px;
}
.inner {
width: 100%;
height: 100%;
background-color: yellow;
/* border: 10px solid red;
border-right: none; */
}
</style>
</head>
<body>
<div class="box1">
<div class="inner"></div>
</div>
</body>
</html>
Margin
<!doctype html>
<html>
<head>
<style>
/*
The margin does not influence the size of visualization area, but the position will be changed.
margin-top
margin-right
margin-bottom
margin-left
margin could be a negative value that the element will move along with the opposite direction.
The abbreviation of margin keep the same with border.
margin will change the area that the box occupy.
*/
.box1 {
width: 200px;
height: 200px;
background-color: #bfa;
border: 10px solid red;
border-right: none;
padding: 20px;
}
.inner {
width: 100%;
height: 100%;
background-color: yellow;
/* border: 10px solid red;
border-right: none; */
}
</style>
</head>
<body>
<div class="box1">
<div class="inner"></div>
</div>
</body>
</html>
Horizontal Layout
<!doctype html>
<html>
<head>
<style>
/*
The horizontal layout
The horizontal layout is defined by the following attributes
margin-left
border-left
padding-left
width
padding-right
boder-right
margin-right
elements in a parent element, the horizontal layer must:
parent width =
margin-left +
border-left +
padding-left +
width +
margin-right +
border-right +
padding-right
The browser will adjust automatically, a value will add to the right side to fill the equation.
若等式不成立,则浏览器会自动调整,在其右侧加上对应差值以使得等式成立
调整情况:
若值中没有auto情况,则浏览器会自动调整margin-right值以使等式满足,
以上值中其中由三个值可以设置为auto
width/ margin-left/ margin-right
-若某个值为auto,则会自动调整auto那个值,以使等式成立
*/
.outer {
width: 800px;
height: 200px;
border: 10px solid red;
}
.inner {
width: 200px;
height: 200px;
background-color: yellow;
}
</style>
</head>
<body>
<div class="outer">
<div class="inner"></div>
</div>
</body>
</html>
Margins Overlap
<!doctype html>
<html>
<head>
<style>
/*
The margin on vertical direction will overlap
Sibbling elements: The bigger value will be selected.
If the value is negative and positive, the sum of the two values
if the two values are both negative, the bigger of the absolute values is selected.
兄弟元素之间的外边距重叠, 对于开发有利,不需要进行处理
父子元素:
- 父子元素相邻外边距,子元素会传递给父元素(上外边距)
- 父子外边距的折叠会影响到页面的布局,必须进行处理
行内元素的盒模型:
-- 行内元素不支持设置宽度和高度
- 行内元素可以设置padding,但是垂直方向padding不会影响页面的布局
- 行内元素border不会影响页面布局
- 行内元素margin不会影响页面布局
display 用来设置元素显示的类型:
可选值:
inline 将元素设置为行内元素
block 将元素设置为块元素
inline-block 将元素设置为行内块元素
行内块,既可以设置高度和宽度又不会独占一行
table 将元素设置为一个表格
none 将元素不在页面中显示
visibility 用来设置元素的显示状态
可选值:
visible 默认值,元素在页面中正常显示
hidden 元素在页面中隐藏,不显示,但依然占据页面位置
*/
.box1, .box2{
width: 200px;
height: 200px
}
.box1 {
background-color: red;
margin-bottom: 50px;
}
.box2 {
background-color: yellow;
margin-top: 30px;
}
.box3 {
width: 100px;
height: 100px;
background-color: #bfa;
border-top: 1px #bfa solid;
padding-top: 100px;
}
</style>
</head>
<body>
<div class="box1">
<div class="box3"></div>
</div>
<div class="box2"></div>
</body>
</html>
Default style
/*
常用的清空默认样式库:
normalize.css: 对默认样式进行了统一
reset.css : 直接去除了浏览器的默认样式
*/
* {
margin: 0px;
padding: 0px;
}